Wow, I thought I had written this up somewhere, but because Ken asked, and he is doing cool stuff with his Connected Course…
Can anyone share the code for the Magic Box #CCourses http://t.co/lv3QFzS45M @cogdog @mburtis @timmmmyboy ?
— Ken Bauer (@ken_bauer) February 11, 2015
What is the Magic Box? Not much magic, but it is meant for the WordPress / Feedwordpress type aggregated sites I have been building the last few years (see my how to series). In the more recent iterations, these sites have a signup form powered by Gravity Forms and custom code that automatically adds people’s blog feeds to the site’s Feed WordPress data.
The Magic Box was something I added to help people figure out their RSS feeds, using some code that fetches the source of that URL and examines it for tell tale signs of RSS (meta tags). It works best on main blog URLs, getting feeds from category and other assorted partial bits of blogs is problematic (that’s a whole other blog post, in the RSS is Great and Messy at the Same Time category).
So typically I link to the Magic Box in the place where we ask for a blog’s RSS feed
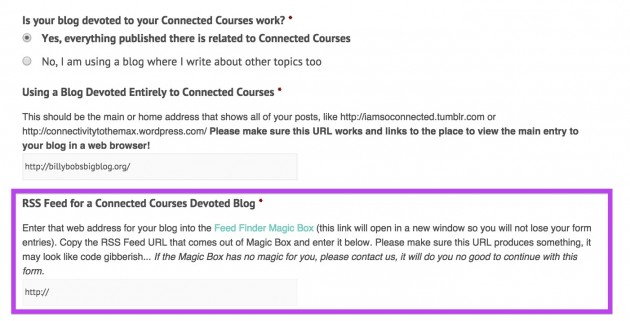
I first made a magic box for the VCU Thought Vectors course, then Connected Courses, Networked Scholars 2014, and most recently for the You Show gig I am doing here at TRU.
They all do the same thing, and you can use them any time. If I knew I was going to keep using it, I might have been smart and hosted it somewhere and just linked as needed, but it’s nice to have it within the navigation of the host course site.
Enough Intro, Alan, How do I make One?
NOTE: Having some issues with the plugin that formats code- get all of the code fragments shown below, you can download as text file.
If I was a bit more deft, I might do it as a plugin, but I don’t see a sign of that happening. So to make one you have to be able to edit your theme’s template files.
These first bits need to go in your functions.php
file. The first function cdb_parameter_queryvars
lets me pass parameters to my templates via the URl string, in our case, the value of the URL field in the form.
The second function feedSearch
actually requests the HTML source of the web site represented by $url
and more or less brute forces looking for the link tags that contain RSS feed addresses. Some sites will have several but in my experience the primary feed is first (wobbly assumption).
The funny thing is parts of this came from code by Martin Hawksey that he attributed back to me and Martha Burtis on the ds106 site. Some sort of boomerang attribution.
[sourcecode lang=”php”]
/* —– add allowable url parameter for urls */
add_filter(‘query_vars’, ‘cdb_parameter_queryvars’ );
function cdb_parameter_queryvars( $qvars )
// add parameters to be passed in wordpress query strings
{
$qvars[] = ‘blogurl’; // for passing blog url
return $qvars;
}
/* —– find primary RSS feed for a given blog (all posts only) */
function feedSearch( $url ) {
// via Martin Hawksey via from me (@cogdog)
if ( $html = @DOMDocument::loadHTML( file_get_contents( $url ) ) ) {
$xpath = new DOMXPath($html);
$results = array();
// First look for RSS 2.0 feeds
$feeds = $xpath->query(“//head/link[@href][@type=’application/rss+xml’]/@href”);
foreach($feeds as $feed) {
$results[] = $feed->nodeValue;
}
// Next first for Atom feeds
$feeds = $xpath->query(“//head/link[@href][@type=’application/atom+xml’]/@href”);
foreach($feeds as $feed) {
$results[] = $feed->nodeValue;
}
// we have somethng
if ( count( $results ) ) {
// we assume (badly) that the first result is the blog feed
return $results[0];
} else {
//oops return an error code
return -1;
}
} else {
// another error if we are unable to get the source HTML
return -1;
}
}
[/sourcecode]
Next you need to create a new WordPress Page that will host the form, name it whatever you like, but make sure it’s slug (the URL field) is exactly magic-box
You can put what ever you like in for some introductory content, and add images. The Magic Box functionality is added via the template code below.
Save that page. It does nothing, yet. We have not added any magic.
Now what you want to do is to look for a file in your WordPress theme called page.php
which is the general template for any WordPress page. Make a copy of that and save it as page-magic-box.php
. This takes advantage of the WordPress Template Hierarchy for Pages.
Our new template will be used only for a page with a slug name of magic-box
.
This first bit of code gets executed before we do any thing for the page layout, it should appear before your template’s code for
It does all the logic when someone submits the form- it makes sure there is something entered, and then sends the value to the feedSearch
function. if it gets something back, it sets up appropriate feedback response.
[sourcecode lang=”php”]
<?php
/* —————————————————————————-
magic box page template
Used to create form to look for RSS feeds
*/
// no feedback for now.
$feedback_msg = '';
// verify that a form was submitted and it passes the nonce check
if ( isset( $_POST['cdb_form_add_feedfinder_submitted'] ) && wp_verify_nonce( $_POST['cdb_form_add_feedfinder_submitted'], 'cdb_form_add_feedfinder' ) ) {
// grab the url from the form
$blogurl = esc_url( trim($_POST['blogurl']), array('http', 'https') );
// let's do some validation, store an error message for each problem found
$errors = array();
if ( empty( $blogurl ) ) {
// missing value for url
$errors[] = 'Blog URL missing or invalid – please enter the full URL for your blog. Please test it to make sure it works’;
} elseif ( strpos( $blogurl, ‘/feed’ ) ) {
$errors[] = ‘Is that really your blog’s web address? It looks like you entered the URL an RSS Feed in the box. Please enter the web address where you blog shows up, the URL that shows your site in a browser.’;
} else {
// see if we can find a feed
$blogrss = feedSearch( $blogurl );
// feed not found by the function
if ($blogrss == -1) $errors[] = ‘Blog feed cound not be found. Make sure the URL that you typed is the one that displays your web site in a browser. Or maybe it is a web site we cannot work magic on. Please check, edit, and try again, or seek assistance (need link).’;
}
if ( count($errors) > 0 ) {
// form errors, build feedback string to display the errors
$feedback_msg = ‘Sorry but the Magic Box cannot process your request:
- ‘;
- ‘ . $oops . ‘
// Hah, each one is an oops, get it?
foreach ($errors as $oops) {
$feedback_msg .= ‘
‘;
}
$feedback_msg .= ‘
‘;
} else {
$feedback_msg = ‘
' . $blogrss . '
You should first verify the URL (opens in a new window) — it will show you something like may look like gibberish, but it represents the raw source of information from your blog and in there you should see remnants of your own blog posts. It should show you something like this or maybe this (depending on your browser).
Make sure you copy this RSS Feed URL to a place where you can find it again.
‘;
}
}
[/sourcecode]
The rest of this comes after the part of your theme that has something like, and finally echoes the appropriate response.
This is the part that puts in the contents of what every you edited in the Page contents (e.g. the instructions for the box). We then insert a small web form to enter a URL. The wp_nonce_field
nonsense is something WordPress does to make sure it only responds to forms submitted from this site (I think).
[sourcecode lang=”php”]
<?php if ( !empty( $feedback_msg ) and !(count($errors)) ) echo '
Success! We have found an RSS Feed for you.
‘;?>
[/sourcecode]
Yes there is probably 500 hundred ways to code this better. But it has worked for me plenty of times. I can certainly hoist it on a site somewhere if someone might find use for it (e.g. it could be used as a link or even iframe for any web site, not just wordpress).
Yeah, I should do that.
Top / Featured Image Credit: Wikimedia Commons image by Shawn from Airdrie, Canada (The magic box) [CC BY-SA 2.0 (http://creativecommons.org/licenses/by-sa/2.0)], via Wikimedia Commons
You truly amaze me with your sharing level and style. Perhaps I can do my part by coding this into a plugin.
I mucked about a bit to get it to play happy with the Beaver Builder child theme but it is almost there.
A note and a question:
#1 There are some spaces between the < and ? on lines 11 and 13 on the form that breaks things. Also something on line 3 with the php call to get the blogurl so I just set that to empty string
#2 How do you get the blue box to show up say on http://youshow.trubox.ca/magic-box/ after submitting a URL? Is that in the css?
Mine just dumps the message up at the top after I figured out where to place the output.
See: http://ken.baueralonso.com/flippedlearning/magic-box/
I tried to do this too quickly!
(#1) Something in the plugin that formats the code is inserting extraneous blanks. Will sort it out or just put on github. Line 3 is critical, and the form will not work of you set blog URL to empty. That is what fetches the value from the form.
(#2) Yes, I use some CSS to generate the boxes.
Will email code.